Blackjack Python 3
This first thing we need to do is create a deck of cards. In order to make a deck of cards we must first understand what a deck of cards is.
A pack is made up of 52 cards (excluding jokers)
Each card in the deck has three attributes:
- Its name
- 1,2,3,4,5,6,7,8,9,10,jack,queen,king
- Its value:
- 1,2,3,4,5,6,7,8,9,10,11,12,13
- Its suit:
- Spades, Diamonds,Hearts,Clubs.
A simple Blackjack game project coded in Python 3.8.
- In Python it is easy to create this full deck of cards using 2 lists and a couple of for loops: Here we have created a list of 52 cards, with each card consisting of a tuple of 2 values: (name,suit).
- In this course, you will learn how to create a Blackjack game by using Python 3. This is meant to be a fun game, an exercise that can be completed during your weekend. This game will randomly assign cards to the player and dealer. This game will also evaluate if either the player or dealer has a Blackjack. The game will then accept player’s input if he wants to draw additional cards.
In Python it is easy to create this full deck of cards using 2 lists and a couple of for loops:
Here we have created a list of 52 cards, with each card consisting of a tuple of 2 values:
(name,suit)
Once we have created the deck of cards it is useful to create a few helper functions to deal with the pack.
- getRealName – This returns the card’s full name in a human readable form
- getValue – This return’s the card’s value, useful for calculations
Creating the mainScreen
In order to create the main game screen we first need to come up with a plan! You can have a look at my plan to the right of the screen.
From the plan I can see that I am going to need:
Functions
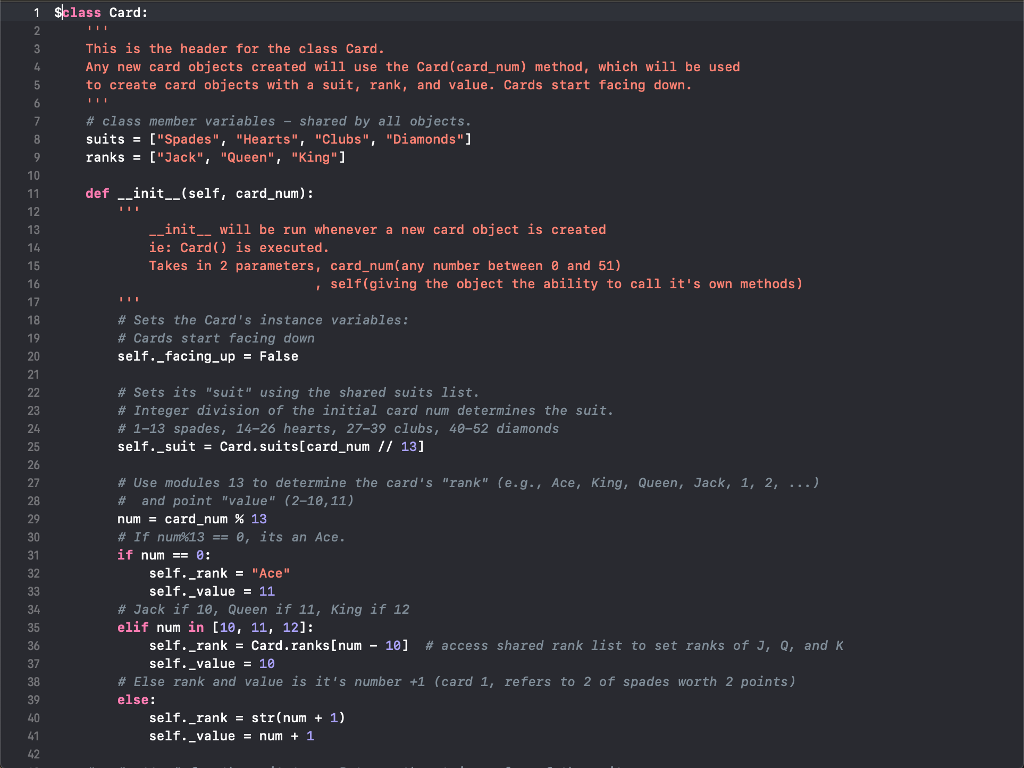
- A MainScreen() function
- A function to display whose turn it is
- A function to display each player’s hand
- A function to get input from the player
- A function to calculate & display who is the winner / loser.
- A function to calculate a player’s score.
Variables

- playerTurn variable to keep track of whose turn it is.
- A round variable to see if it is round one or 2.
Data Structures
- A list for each player to contain the cards that they have been dealt.
Do you want to learn Python? What better way is there than to learn it by having a fun project?
Blackjack Python 3 Cheat

In this course, you will learn how to create a Blackjack game by using Python 3. This is meant to be a fun game, an exercise that can be completed during your weekend.
This game will randomly assign cards to the player and dealer. This game will also evaluate if either the player or dealer has a Blackjack. The game will then accept player’s input if he wants to draw additional cards. The dealer will then draw his cards. The game ends by checking who has a better hand.

Blackjack Python 3 Free
In this course, we will be going through some key concepts, such as list, dictionary, functions, and loops.
This is a hands-on course. You will be coding along side with me. I’ll be guiding you step-by-step along the way. Jupyter notebooks are included at each lesson to further reinforce your understanding on the particular topics.
You don’t need to know Python, as this course is made for a beginner. Even if you know some basic, you can still get along with the course.
If you are interested in learning this course, I’ll see you on the inside.
Happy learning!